Create Sendable Type for non-Sendable Types in Swift Concurrency
Learn how to create Sendable types from non-Sendable types when adopting Swift 6 concurrency. Discover strategies using actors, wrappers with locks, and the @unchecked Sendable attribute with code examples.
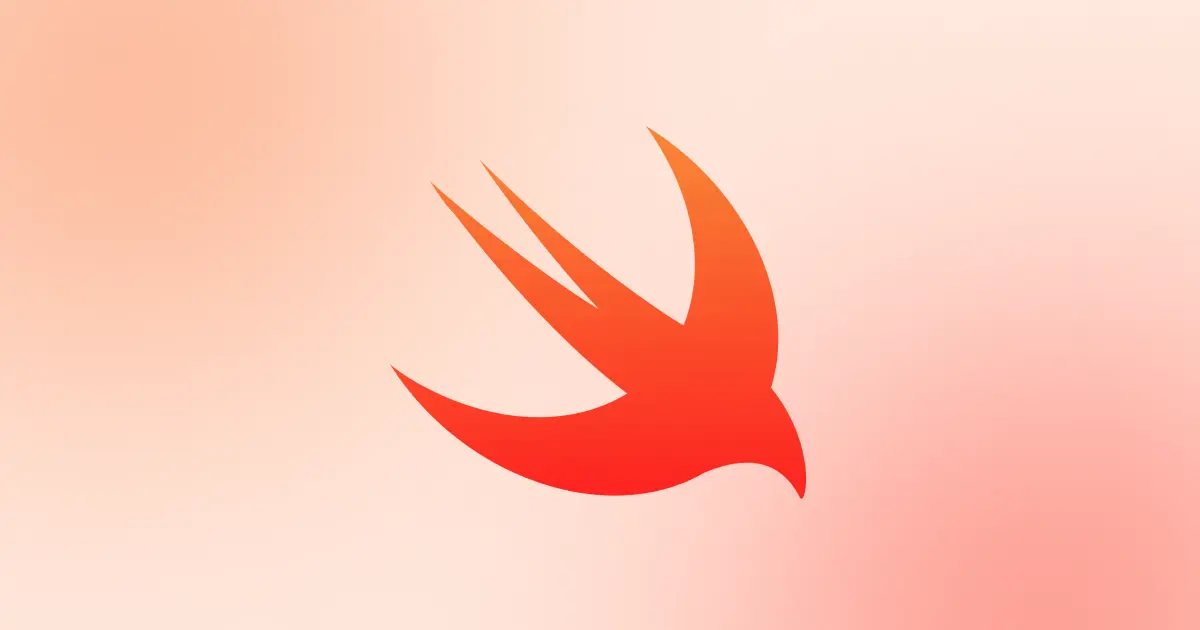
Earlier this year, I completed the Practical Swift Concurrency book by Donny Wals. It was a great book but still not fully updated with Swift 6. So when I made the jump and activate complete concurrency and Swift 6 in my side project, I was greeted with hundreds of errors and there was no resource that I can reference to. Most of the errors were because I send a non-Sendable to concurrent code. So I decided to write this blog post to help you (and my future self!) to make the transition to Swift 6 easier.
Enable concurrency checking
To follow the tutorial, you must set the “String Concurrency Checking” to “Complete” and change the “Swift Language Version” to “Swift 6” in your build settings. You can follow this documentation from Apple if you are feeling lost.
Once you are done, you can check whether you have set up everything correctly by copy and paste this code to your project:
@Observableclass ViewModel {
private(set) var image: UIImage?
func onAppear() { Task { [weak self] in guard let self else { return } self.image = await fetchImage() } }
private func fetchImage() async -> UIImage { // TODO: call our repository to fetch the image return UIImage() }
}
If you get error “Passing closure as a ‘sending’ parameter risks causing data races between code in the current task and concurrent execution of the closure”, it means you have set up everything correctly.
If you get warning or nothing at all, it means you have not set up the project correctly.
How to create a Sendable from a non-Sendable type
We have some options on how to make a non-Sendable type Sendable. When I need to create a Sendable from a non-Sendable type, it usually depends on whether I need to change the properties of the type after we create it or not. In this blog post, we will discuss those options one by one.
When we don’t modify the properties of the type after we create it (constants).
If we don’t need to modify the properties of the type after you create it, I found there are 3 options:
Using actors with constant properties
We can wrap the non-Sendable type with an actor, and make it a constant. Actors are Sendable by default because actors provide data isolation, meaning that they ensure only one task at a time can access their data.
actor ImageActor { let image: UIImage
init(image: UIImage) { self.image = image }}
Actors comes with a little inconvenience though. Since actors protect their
data, if you do something that may change the state of the actor from
outside of actor’s context, you have to await
the operation to ensure
exclusive access to its mutable state. In this example, since our image
is
using let
, the compiler is smart enough to know that we won’t change the
image. So we don’t need to await
to read from image:
let imageActor = ImageActor(image: UIImage())let image = imageActor.image // no error
If you change the image
to var
, you will get an error “Actor-isolated
property ‘image’ can not be referenced from a nonisolated context”.
Use @unchecked Sendable
If you only need the type to be constant value, the most simple, but may not
the correct option is to use the @unchecked Sendable
conformance that looks
like this:
extension UIImage: @unchecked Sendable {}
What @unchecked
does is tells the compiler to trust us that the type is
Sendable. The compiler will not check the conformance for us, so we take the
responsibility to make sure that the type is indeed thread-safe.
In this code, we are telling the compiler that UIImage
is Sendable. But we
don’t do anything to make sure that UIImage
is thread-safe. Depending on
your project, most likely you don’t change the properties of UIImage
after
you create it. So in most cases it’s okay to use @unchecked Sendable
for
UIImage
.
Be aware though as this code may lead to subtle bugs because UIImage
is
not thread-safe, but your teammates or future you may think that it is. So
if you change the properties of UIImage
after you create it, you will get
race conditions.
@unchecked Sendable wrapper with non-sendable constant.
The third option for constant value is to create a wrapper for the non-Sendable type. It looks like this.
/// A thread-safe wrapper around a `UIImage`.////// - Warning: This type should be treated as immutable. You can read from it, but DO NOT write to it.final class FinalImage: @unchecked Sendable {
/// - Warning: You can read from it, but DO NOT write to it as it's not thread safe. let image: UIImage
init(image: UIImage) { self.image = image }
}
This approach is better compared to the second approach because now we are
using a wrapper instead of UIImage
directly. So we can tell our teammates
that we should not change the properties of FinalImage
after we create it.
When we need to modify the properties of the type after we create it (mutable state).
Using Actors
The previous 3 options are only suitable for constant values. If your type
have a mutable state, you can’t make it conform to Sendable. One of the
option is to use actor
. Consider the following code:
final class ImageLoader {
private var cacheImages = [String: UIImage]()
func load(key: String) async -> UIImage { if let image = cacheImages[key] { return image }
let image = await loadFromDisk(key: key) cacheImages[key] = image return image }
private func loadFromDisk(key: String) async -> UIImage { // load from disk return UIImage() }
}
Everytime you call load(key:)
method, you may change the state of
cacheImages
. So if you do it concurrently, you will have race condition.
You can solve this by using actor
to protect the mutable state. To change
it to an actor
you just need to change the class
to actor
:
actor ImageLoader {
16 collapsed lines
private var cacheImages = [String: UIImage]()
func load(key: String) async -> UIImage { if let image = cacheImages[key] { return image }
let image = await loadFromDisk(key: key) cacheImages[key] = image return image }
private func loadFromDisk(key: String) async -> UIImage { // load from disk return UIImage() }
}
Wrapper with locks for every Operation
In my project, I need a Sendable version of CurrentValueSubject
.
As you may already know, CurrentValueSubject
have a mutable state that
changed everytime you call send(_:)
method. We can use actors like the
previous example, but for this I don’t want to introduce await
to its
methods. So I create a wrapper with locks that looks like this:
import Combine
final class ThreadSafeCurrentValueSubject<Output, Failure: Error>: @unchecked Sendable {
private let currentValueSubject: CurrentValueSubject<Output, Failure> private let lock = NSLock()
var value: Output { currentValueSubject.value }
init(_ value: Output) { self.currentValueSubject = CurrentValueSubject(value) }
func send(_ input: Output) { lock.lock() defer { lock.unlock() } currentValueSubject.send(input) }
func completion(_ completion: Subscribers.Completion<Failure>) { lock.lock() defer { lock.unlock() } currentValueSubject.send(completion: completion) }
}
The usage of this identical to CurrentValueSubject
, but now it’s
thread-safe. We protect the mutable state by using NSLock
for every operation
that change the mutable state. We unlock the lock using defer
to ensure we
always call unlock
once we are done.
Wrapper with a method to modify mutable state
You may have a type that have mutable states. Sure you can use actor
, but
sometime having to use await
is not an option. You can make it thread-safe by
provide a single method to modify the state using locks. Let’s say you have
this:
class Foo { var bar = 0}
You can’t just make it Sendable because it has a mutable state of bar
. So
we will need to make it conforms to Sendable manually using @unchecked Sendable
.
To solve this, you can change the bar
to be read only, and create an
underlying state that is private. I will name it _bar
final class Foo: @unchecked Sendable {
var bar = 0 var bar: Int { _bar }
private var _bar = 0
}
Now, we can provide a method to modify the state of bar
using locks:
final class Foo: @unchecked Sendable {
7 collapsed lines
private let lock = NSLock()
var bar: Int { _bar }
private var _bar = 0
func modify(modifier: (inout Int) -> Void) { lock.lock() modifier(&_bar) lock.unlock() }
}
With this, you can modify the state of bar
safely:
let foo = Foo()print("before:", foo.bar) // before: 0
foo.modify { bar in bar = 10}
print("after:", foo.bar) // before: 10
Closing
I hope this blog post helps you to make the transition to Swift 6 easier. If you find another and better way to make a non-Sendable type Sendable, please let me know in the comment below. I would love to discuss!